Laravel Commands
creating new project:
composer create-project --prefer-dist laravel/laravel projectName '5.4.*'
creating middleware:
php artisan make:middleware
https://scotch.io/tutorials/understanding-laravel-middleware
Create Table on Database:
php artisan make:migration sampleTable
php artisan make:migration create_users_table
Change column type on table
php artisan make:migration change_completion_status_datatype_in_donation_product_backups_table
public function up(): void
{
Schema::table('week_features', function (Blueprint $table) {
$table->text('description')->change();
});
}
/**
* Reverse the migrations.
*/
public function down(): void
{
Schema::table('week_features', function (Blueprint $table) {
$table->string('description')->change();
});
}
Add new columns to table:
php artisan make:migration add_phone_to_sampleTable --table="users"
execute table creation:
php artisan migrate
Undo migration recent:
php artisan migrate:rollback --step=1
Using laravel Authentication with password reset:
https://tutorials.kode-blog.com/laravel-authentication-with-password-reset
create user auth with role:
https://www.kerneldev.com/2018/02/12/set-up-role-based-access-control-in-laravel/
installing additional package:
sample: composer require vinkla/hashids
issue: method not exist
solution: composer dump-autoload -o
issue: TokenMismatchException
put {{ csrf_field() }} inside form
issue: laravel Class 'App\Http\Controllers\Input' not found
https://stackoverflow.com/questions/31696679/laravel-5-class-input-not-found
https://laracasts.com/discuss/channels/general-discussion/l5-apphttpcontrollersinput-not-found?page=1
create user:
https://blog.indiefolk.co.uk/laravel-4-create-users-with-artisan-tinker/
create controller:
php artisan make:controller ControllerName
php artisan make:controller ControllerName --resource //make controller as resource for CRUD
Create Controller and Model
php artisan make:controller TodoController --resource --model=Todo
Create Model with migration
php artisan make:model ModelName -m
Create Model, controller, factory
php artisan make:model Todo -a
— output —
INFO Model [app/Models/Blog.php] created successfully.
INFO Factory [database/factories/BlogFactory.php] created successfully.
INFO Migration [database/migrations/2024_08_12_211451_create_blogs_table.php] created successfully.
INFO Seeder [database/seeders/BlogSeeder.php] created successfully.
INFO Request [app/Http/Requests/StoreBlogRequest.php] created successfully.
INFO Request [app/Http/Requests/UpdateBlogRequest.php] created successfully.
INFO Controller [app/Http/Controllers/BlogController.php] created successfully.
INFO Policy [app/Policies/BlogPolicy.php] created successfully.
——
Create controller with API:
Php artisan make:controller ControllwerName —api
create model:
php artisan make:model ModelName
create json error response:
https://stackoverflow.com/questions/22414524/how-can-i-make-laravel-return-a-custom-error-for-a-json-rest-api
install yajra datatables laravel:
http://yajrabox.com/docs/laravel-datatables/master/installation
composer require yajra/laravel-datatables-oracle:^8.0
issue: https://github.com/yajra/laravel-datatables/issues/1490
publishing vendor
php artisan vendor:publish
check laravel version:
php artisan laravel --version
Hashids:
https://github.com/Torann/laravel-hashids
deploying laravel application on VPS with VestaCp panel
------------------------------------------------------
http://www.fargowebdesign.com/2016/03/02/deploying-a-laravel-application/
if open_basedir error: edit /home/admn/conf/web/[webdomain].httpd.conf and add the path of laravel app to the open_basedir value
issue: Specified key was too long; max key length is 767 in php artisan migrate
solution: https://laravel-news.com/laravel-5-4-key-too-long-error
install hashids library
composer require hashids/hashids
Install Sortable table
composer require spatie/eloquent-sortable
Install Yajra datatable
composer require yajra/laravel-datatables-oracle
Get Laravel version:
Php artisan —version
link storage to public
php artisan storage:link
Display route list
php artisan route:list
Clear View Cache
php artisan view:cache
php artisan view:clear
composer dump
php artisan route:cache
php artisan route:clear
Make Middleware
php artisan make:middleware EnsureTokenIsValid
List all laravel commands
php artisan list
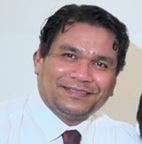
Dario L. Mindoro
Author of Mindworksoft.com, Full-stack developer, interested in media streaming, automation, photography, AI, and digital electronics.