Create a C# app for Salary Calculator
Here is my simple desktop application created in C#, this is just to show how simple it is to program in C# using Visual Studio 2019, the Calculator app was inspired by the online version from Calculator.net . As I am confident in the C style language which is the same style used in Java, C++, Javascript, and all those languages that its codes end with a semi-colon, creating this app is very easy.
This is the snapshot of the app, the user interface is pretty simple some input parameters on the left and the output data on the right, processing take place once the Calculate button is clicked.
To start with the project here are some steps to do, please note that you need to have a Visual Studio 2019 installed on your computer otherwise you can download it from the Microsoft website, I am using the FREE version which is the community version of Visual Studio.
1. Open Visual Studio and create new project by clicking the File -> new - project
2. On the next dialog choose new project
3. On the next dialog select Windows Form App (.NET Framework). This is normally used for creating an application for Windows desktop
4. Click next and on the last steps is to configure your project name, location, and other setups, you may leave the other as default so only change the Project name and location only.
5. User interface layout. This is where you can just arrange items on the form, you can click and expand the toolbox on the left side of the Visual Studio IDE to select several items such as buttons, textboxes, combo boxes, and labels, here below my layout you can follow it or create your own, please note that you need to name properly those controls that is being used on the codes such as the salary amount textbox because you code will access the value on this control, you can specify the name of every control on the property window and the right bottom area of the IDE. You can actually run the app by clicking the start button on the top menu of IDE to see how it looks on the actual executable window although it does nothing at all.
6. Start coding. Once you've done with the layout and naming the controls you can now start coding to make the app functional,
On my app I named the Calculate button as btnCalculate and when you double click on it the IDE will show you the code window showing the function event that will be execute any code with in it, the function code look like below.
Form1.cs
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using System.Diagnostics; namespace Salary_Calculator { public partial class frmMain : Form { public frmMain() { InitializeComponent(); } private void frmMain_Load(object sender, EventArgs e) { } private void btnCalculate_Click(object sender, EventArgs e) { } } }
This is the whole code for the form, it start with the Class and a method inside it, you can also see the frmMain() method this is where the app execute any code on it when the app initializes, then you'll see the btnCalculate_Click so that is the method to execute when you click the button on it.
7. Make use of the OOP.
You can put all your codes on it for the app to execute but to make the codes more manageable you need to make use of the OOP style, so I create a new Class for the this app called Salary class, you can do this by right-clicking the solution explorer on the right side panel select add - > new item.
On the new item dialog select Class and specify the class name you like, in my case I use Salary as the class name, this will be the file name and the class name as well.
A new file will be created and you will see a class template that is ready for you to add codes on it.
Salary.cs
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace Salary_Calculator { class Salary { } }
Here below are part of my codes for the Salary class so you may add or finish it improve it if you like
Salary.cs
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace Salary_Calculator { class Salary { int hoursPerWeek; int daysPerWeek; int holidaysPerYear; int vacationPerYear; double salaryAmount; string perFrequent; public double unHourly; public double unDaily; public double unWeekly; public double unBiWeekly; public double unSemiMonthly; public double unMonthly; public double unQuarterly; public double unAnnual; public double Hourly; public double Daily; public double Weekly; public double BiWeekly; public double SemiMonthly; public double Monthly; public double Quarterly; public double Annual; public Salary(double _salaryAmount=25,string _perFrequent="Hour") { salaryAmount = _salaryAmount; perFrequent = _perFrequent; //default values; hoursPerWeek = 40; daysPerWeek = 5; holidaysPerYear = 10; vacationPerYear = 15; } public void setHoursPerWeek(int _hoursPerWeek) { hoursPerWeek = _hoursPerWeek; } public int getHoursPerWeek() { return hoursPerWeek; } public void setDaysPerWeek(int _daysPerWeek) { daysPerWeek = _daysPerWeek; } public int getDaysPerWeek() { return daysPerWeek; } public void setHolidaysPerYear(int _holidaysPerYear) { holidaysPerYear = _holidaysPerYear; } public int getHolidaysPerYear() { return holidaysPerYear; } public void setVacationPerYear(int _vacationPerYear) { vacationPerYear = _vacationPerYear; } public int getVacationPerYear() { return vacationPerYear; } public void setSalaryAmount(double _salaryAmount) { salaryAmount = _salaryAmount; } public void setPerFrequent(string _perFrequent) { perFrequent = _perFrequent; } public void calculate() { unHourly = salaryAmount; unDaily = salaryAmount*8; //8 hours a day unWeekly = salaryAmount * hoursPerWeek; unBiWeekly = unWeekly * 2; } } }
And on the main form here below my codes, please note that I intentionally put just the half of the codes for you to explore and improve so you will learn from it.
Form1.cs
//-------------------------------------------------------- // Program to compute Salary base on the given parameters // Author: Dario Mindoro - www.mindworksoft.com //-------------------------------------------------------- using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using System.Diagnostics; namespace Salary_Calculator { public partial class frmMain : Form { Salary sc; public frmMain() { InitializeComponent(); } private void frmMain_Load(object sender, EventArgs e) { DictionarycomboSource = new Dictionary (); comboSource.Add(0, "Hour"); comboSource.Add(1, "Day"); comboSource.Add(2, "Week"); comboSource.Add(3, "Bi-Week"); comboSource.Add(4, "Semi-Month"); comboSource.Add(5, "Month"); comboSource.Add(6, "Quarter"); comboSource.Add(7, "Year"); cmbPer.DataSource = new BindingSource(comboSource, null); cmbPer.DisplayMember = "Value"; cmbPer.ValueMember = "Key"; cmbPer.SelectedIndex = 0; txtSalaryAmount.Text = "0"; sc = new Salary(); txtHourPerWeek.Text = sc.getHoursPerWeek().ToString(); txtDaysPerWeek.Text = sc.getDaysPerWeek().ToString(); txtHolidaysPerYear.Text = sc.getHolidaysPerYear().ToString(); txtVacationPerYear.Text = sc.getVacationPerYear().ToString(); } private void btnCalculate_Click(object sender, EventArgs e) { sc.setSalaryAmount(double.Parse(txtSalaryAmount.Text)); sc.calculate(); lblUnDaily.Text = String.Format("{0:C}", Convert.ToInt32(sc.unDaily)); lblUnHourly.Text = String.Format("{0:C}", Convert.ToInt32(sc.unHourly)); lblUnWeekly.Text = String.Format("{0:C}", Convert.ToInt32(sc.unWeekly)); lblUnBiWeekly.Text = String.Format("{0:C}", Convert.ToInt32(sc.unBiWeekly)); } } }
Conclusion
This concludes my tutorial on creating a simple C# application, you can actually take the executable file on the Bin folder from your project folder so you can run your app without the Visual Studio IDE, but if you wish to run the app on the computer without the Visual Studio you need to create an Installer for it so it will go along with the required libraries in order to run as a standalone app.
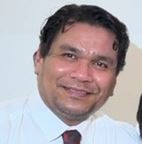
Dario Mindoro
Author of Mindworksoft.com, Full-stack developer, interested in media streaming, automation, photography, AI, and digital electronics